ANSYS::Nexus::GLTFWriter::Scene Class Reference
Last update: 17.04.2023Scenes are the GLTFWriter class that create the view of the data that is defined in the GLTF file. More...
#include <GLTFWriter.h>
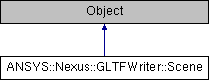
Public Types | |
enum | BackgroundType { BT_NONE = -1 , BT_SOLID = 0 , BT_TB , BT_LR , BT_TLBR } |
Public Member Functions | |
virtual bool | SetCamera (Node *camera)=0 |
virtual bool | SetLight (Node *light)=0 |
virtual bool | SetProxyImage (Node *image)=0 |
virtual void | SetClipPlane (float p0x, float p0y, float p0z, float p1x, float p1y, float p1z, float p2x, float p2y, float p2z)=0 |
virtual bool | AppendMesh (Node *mesh)=0 |
virtual bool | Add2DText (GLTF *gltf, const char *text, float x, float y, Markup::MarkupTextAttachmentType textAttach=Markup::MTAT_CENTER, float textSize=20, const char *textFont=0, float textR=0, float textG=0, float textB=0, float bgR=1, float bgG=1, float bgB=1, float bgA=0, const char *path=0)=0 |
virtual bool | Add3DText (GLTF *gltf, const char *text, float x, float y, float z, Markup::MarkupTextAttachmentType textAttach=Markup::MTAT_AUTO, float textSize=12, const char *textFont=0, float textOffX=0, float textOffY=0, Markup::MarkupLineType lineShape=Markup::MLT_LINE, float lineR=0, float lineG=0, float lineB=0, float textR=0, float textG=0, float textB=0, float bgR=1, float bgG=1, float bgB=1, float bgA=0.5, const char *path=0)=0 |
Static Public Member Functions | |
static Scene * | Create (GLTF *gltf, const char *name=0, const char *units=0, float scale=1, Scene::BackgroundType backgroundType=Scene::BT_NONE, float r1=0, float g1=0, float b1=0, float r2=0, float g2=0, float b2=0) |
Detailed Description
Scenes are the GLTFWriter class that create the view of the data that is defined in the GLTF file.
Multiple scene can be defined, but only one is visible at a time. The initial scene displayed is defined by SetDefaultScene
Definition at line 28 of file GLTFScene.h.
Member Enumeration Documentation
◆ BackgroundType
Type of background.
Definition at line 32 of file GLTFScene.h.
Member Function Documentation
◆ Add2DText()
|
pure virtual |
Adds a 2D text label to the scene. 2D text labels remain stationary when the viewer is transformed.
- Returns
- Status of Add2DText.
- Parameters
-
[in] gltf GLTF object. [in] text Text label. [in] x Horizontal offset from left. Ranges from 0 to 1. [in] y Vertical offset from top. Ranges from 0 to 1. [in] textAttach Optional, Markup::MarkupTextAttachmentType describing how label is positioned relative to x,y. [in] textSize Optional, height of label font in pixels. [in] textFont Optional, label font. Good choices are "serif" and "sans-serif". [in] textR Optional, red component of text color. Ranges from 0 to 1. [in] textG Optional, green component of text color. Ranges from 0 to 1. [in] textB Optional, blue component of text color. Ranges from 0 to 1. [in] bgR Optional, red component of text background. Ranges from 0 to 1. [in] bgG Optional, green component of text background. Ranges from 0 to 1. [in] bgB Optional, blue component of text background. Ranges from 0 to 1. [in] bgA Optional, alpha component of text background. Ranges from 0 to 1. [in] path Optional full path to node to which this markup is attached. If not specified, markup is attached to scene. If specified, the markup does not appear in the visibility selector under annotations. The visibility is controlled by the node's visibility. For a node that is directly under the scene, this is simply the Node ID of the node. For a Node that is a child of another node, the path is "NodeIDParent;NodeID". The path is simply a concatenation of nodeIDs that lead to the node to which the markup is attached. The concatenation is with the ';' character.
◆ Add3DText()
|
pure virtual |
Adds a 3D text label to the scene. 3D text labels change position when the viewer is transformed.
- Returns
- Status of Add3DText.
- Parameters
-
[in] gltf GLTF object. [in] text Text label. [in] x X location in mesh space. [in] y Y location in mesh space. [in] z Z location in mesh space. [in] textAttach Optional, Markup::MarkupTextAttachmentType describing how label is positioned relative to x,y,z + textOffX,textOffY. [in] textSize Optional, height of label font in pixels. [in] textFont Optional, label font. Good choices are "serif" and "sans-serif". [in] textOffX Optional amount label is offset, in pixels, from x,y,z in screen X direction. [in] textOffY Optional amount label is offset, in pixels, from x,y,z in screen Y direction. [in] lineShape Optional, Markup::MarkupLineType defining symbol at end of line. [in] lineR Optional red component of line color. Ranges from 0 to 1. [in] lineG Optional green component of line color. Ranges from 0 to 1. [in] lineB Optional blue component of line color. Ranges from 0 to 1. [in] textR Optional red component of text color. Ranges from 0 to 1. [in] textG Optional green component of text color. Ranges from 0 to 1. [in] textB Optional blue component of text color. Ranges from 0 to 1. [in] bgR Optional red component of text background. Ranges from 0 to 1. [in] bgG Optional green component of text background. Ranges from 0 to 1. [in] bgB Optional blue component of text background. Ranges from 0 to 1. [in] bgA Optional alpha component of text background. Ranges from 0 to 1. [in] path Optional full path to node to which this markup is attached. If not specified, markup is attached to scene. If specified, the markup moves with node and visibility is controlled by node, as well as the Annotations entry under the visibility selector. For a node that is directly under the scene, this is simply the Node ID of the node. For a Node that is a child of another node, the path is "NodeIDParent;NodeID". The path is simply a concatenation of nodeIDs that lead to the node to which the markup is attached. The concatenation is with the ';' character.
◆ AppendMesh()
|
pure virtual |
Adds a mesh node to the scene. Multiple mesh nodes can be appended to a scene.
- Returns
- Status of AppendMesh.
- Parameters
-
[in] mesh Mesh node.
◆ Create()
|
static |
Creates a GLTF scene. The scene node is automatically added to the GLTF file.
- Returns
- Scene object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] name Optional, name of scene. [in] units Optional, units to display with ruler. [in] scale Optional, scale factor that converts displayed units to scene mesh units. [in] backgroundType Optional, Scene::BackgroundType defining type of background to create. [in] r1 Optional, red component of solid color or first color of gradient. [in] g1 Optional, green component of solid color or first color of gradient. [in] b1 Optional, blue component of solid color or first color of gradient. [in] r2 Optional, red component of second color of gradient. [in] g2 Optional, green component of second color of gradient. [in] b2 Optional, blue component of second color of gradient.
- Examples
- Test001.cpp, Test002.cpp, Test003.cpp, Test004.cpp, Test005.cpp, Test006.cpp, Test007.cpp, Test008.cpp, Test009.cpp, Test010.cpp, Test011.cpp, Test012.cpp, Test013.cpp, and Test014.cpp.
◆ SetCamera()
|
pure virtual |
Sets the camera node for the scene. Only a single camera can be set to a scene. If this is called a second time the second camera will be attached to the scene. The camera node is optional. By default, an orthographic camera is created with an isometric orientation that encompasses the entire scene.
- Returns
- Status of SetCamera.
- Parameters
-
[in] camera Camera node.
◆ SetClipPlane()
|
pure virtual |
Sets a clip plane based on the three points given. Clipping plane is based on right hand rule, where normal is given by thumb direction when fingers follow direction of three points.
The part of the object above the plane (normal direction) is clipped
- Parameters
-
[in] p0x x coordinate of first point of plane. [in] p0y y coordinate of first point of plane. [in] p0z z coordinate of first point of plane. [in] p1x x coordinate of second point of plane. [in] p1y y coordinate of second point of plane. [in] p1z z coordinate of second point of plane. [in] p2x x coordinate of third point of plane. [in] p2y y coordinate of third point of plane. [in] p2z z coordinate of third point of plane.
◆ SetLight()
|
pure virtual |
Sets the light node for the scene. This node is only required for techniques that have auto-generated programs. All lighting calculations must be done in shader programs.
- Returns
- Status of SetLight.
- Parameters
-
[in] light Light node.
◆ SetProxyImage()
|
pure virtual |
Sets the proxy image node for the scene. A proxy image can be used while loading or on a system that does not support WebGL. The proxy image node is optional.
- Returns
- Status of SetProxyImage.
- Parameters
-
[in] image Image node.
The documentation for this class was generated from the following file:
- D:/ANSYSDev/NoBackup/branches/EnSight-Second-Coming/webgl_viewer/gltfwriterlib/include/GLTFScene.h