ANSYS::Nexus::GLTFWriter::Attribute Class Reference
Last update: 17.04.2023Attributes define the per element index values for elements defined by Index. More...
#include <GLTFWriter.h>
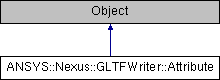
Public Types | |
enum | AttributeType { AT_INT = 5124 , AT_FLOAT = 5126 , AT_FLOAT_VEC2 = 35664 , AT_FLOAT_VEC3 = 35665 , AT_FLOAT_VEC4 = 35666 , AT_FLOAT_MAT4 = 35676 } |
Public Member Functions | |
virtual bool | SetMinMax (AttributeType type, const int *mn, const int *mx)=0 |
virtual bool | SetMinMax (AttributeType type, const float *mn, const float *mx)=0 |
virtual void | SetLogarithmic (bool log)=0 |
Static Public Member Functions | |
static Attribute * | Create (GLTF *gltf, const char *name, AttributeType type, unsigned int count, const int *data, Buffer *buffer=0) |
static Attribute * | Create (GLTF *gltf, const char *name, AttributeType type, unsigned int count, const float *data, Buffer *buffer=0) |
static Attribute * | CreatePosition (GLTF *gltf, unsigned int count, const float *data, Buffer *buffer=0) |
static Attribute * | CreateColor (GLTF *gltf, AttributeType type, unsigned int count, const float *data, Buffer *buffer=0) |
static Attribute * | CreatePointSize (GLTF *gltf, unsigned int count, const float *data, Buffer *buffer=0) |
static Attribute * | CreateDisplacementScale (GLTF *gltf, unsigned int count, const float *data, Buffer *buffer=0) |
static Attribute * | CreateNormal (GLTF *gltf, unsigned int count, const float *data, Buffer *buffer=0) |
static Attribute * | CreateTextureCoord (GLTF *gltf, unsigned int num, unsigned int count, const float *data, Buffer *buffer=0) |
Detailed Description
Attributes define the per element index values for elements defined by Index.
There must be an attributes that defines the vertices to use for the element indices. Other attributes defining colors, normals, texture coordinates, etc., may be defined.
Attributes are part of the primitive and are eventually passed to the vertex shader. All attributes of the vertex shader must have a corresponding attribute defined in the primitive.
Definition at line 31 of file GLTFAttribute.h.
Member Enumeration Documentation
◆ AttributeType
Type of attribute.
Enumerator | |
---|---|
AT_INT | 4 byte integer |
AT_FLOAT | 4 byte float |
AT_FLOAT_VEC2 | 2 AT_FLOATs |
AT_FLOAT_VEC3 | 3 AT_FLOATs |
AT_FLOAT_VEC4 | 4 AT_FLOATs |
AT_FLOAT_MAT4 | 4x4 (16) AT_FLOATs |
Definition at line 35 of file GLTFAttribute.h.
Member Function Documentation
◆ Create() [1/2]
|
static |
Creates an attribute of the given type and name. There are several names that are reserved for special use:
- POSITION - vertices of element index, this attribute must be defined
- TEXCOORD0 - variable value at element index
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] name Name of attribute. [in] type Attribute::AttributeType defining type of attribute. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
◆ Create() [2/2]
|
static |
Creates an attribute of the given type and name. There are several names that are reserved for special use:
- POSITION - vertices of element index, this attribute must be defined
- TEXCOORD0 - variable value at element index
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] name Name of attribute. [in] type Attribute::AttributeType defining type of attribute. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test002.cpp, Test006.cpp, Test008.cpp, and Test013.cpp.
◆ CreateColor()
|
static |
Creates an attribute of name "COLOR". The "COLOR" attribute is usually optional.
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] type Attribute::AttributeType defining type of attribute. [in] count Number of attributes. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test005.cpp.
◆ CreateDisplacementScale()
|
static |
Creates an attribute of type AT_FLOAT_VEC4 and name "DISPLACEMENTSCALE". The "DISPLACEMENTSCALE" attribute is usually optional. It specifies a translation XYZ and a uniform scale S as a four float tuple XYZS
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test014.cpp.
◆ CreateNormal()
|
static |
Creates an attribute of type AT_FLOAT_VEC3 and name "NORMAL". The normal data must be normalized. The "NORMAL" attribute is usually optional. It is required for lighting calculations and spherical texture coordinate mapping.
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test005.cpp, and Test010.cpp.
◆ CreatePointSize()
|
static |
Creates an attribute of type AT_FLOAT and name "POINTSIZE". The "POINTSIZE" attribute is usually optional.
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test009.cpp.
◆ CreatePosition()
|
static |
Creates an attribute of type AT_FLOAT_VEC3 and name "POSITION". Every primitive must have an attribute with the name "POSITION". The GLTF Viewer uses the "POSITION" to perform hit detection and probing.
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test005.cpp, Test009.cpp, Test010.cpp, and Test014.cpp.
◆ CreateTextureCoord()
|
static |
Creates an attribute of type AT_FLOAT_VEC and name "TEXCOORDnum". This creates a texture coordinate of type AT_FLOAT. Use Create to create texture coordinates into 2D textures. Texture coordinates are optional, but generally required for texture mapped materials. If the primitive has a "TEXCOORD0", the texture coordinate must be a 1D texture coordinate, as in the value of results variable. The GLTF Viewer will use this texture coordinate to perform probing. The value at the probe point will be the value of the texture coordinate.
- Returns
- Attribute object. Do not delete this pointer.
- Parameters
-
[in] gltf GLTF object. [in] num Texture coordinate number. [in] count Number of attributes. Number is relative to sizeof type. [in] data Data for attribute. data type is base type of attribute. [in] buffer Optional, Buffer into which to write attribute data. Data is appended to buffer.
- Examples
- Test009.cpp, and Test014.cpp.
◆ SetLogarithmic()
|
pure virtual |
Sets a flag indicating whether to log the data values. Only used for results type data.
- Parameters
-
[in] log log flag.
◆ SetMinMax() [1/2]
|
pure virtual |
Sets the min and max value of an attribute. The min and max value is required for all attributes. If SetMinMax is not called, the min and max values are automatically calculated from the attribute. Under some situations this is not the desired value. When programs are auto-generated, and the primitive has a "TEXCOORD0", the generated program will use the min/max of the texture coordinate to normalize the texture coordinate value for use as a texture lookup value.
- Returns
- Status of SetMinMax.
- Parameters
-
[in] type Attribute::AttributeType defining type of attribute. [in] mn Minimum value of attribute. [in] mx Maximum value of attribute.
◆ SetMinMax() [2/2]
|
pure virtual |
Sets the min and max value of an attribute. The min and max value is required for all attributes. If SetMinMax is not called, the min and max values are automatically calculated from the attribute. Under some situations this is not the desired value. When programs are auto-generated, and the primitive has a "TEXCOORD0", the generated program will use the min/max of the texture coordinate to normalize the texture coordinate value for use as a texture lookup value.
- Returns
- Status of SetMinMax.
- Parameters
-
[in] type Attribute::AttributeType defining type of attribute. [in] mn Minimum value of attribute. [in] mx Maximum value of attribute.
The documentation for this class was generated from the following file:
- D:/ANSYSDev/NoBackup/branches/EnSight-Second-Coming/webgl_viewer/gltfwriterlib/include/GLTFAttribute.h